CSAPP-note-1
Floating Point
Floating Point Representation
Normalized Values
Numerical Form:
- S is a sign bit, it determines whether number is negative or positive.
- Significand M normally a fractional value in range [1.0,2.0).
- E weigths value by power of 2.
1 | Single precision: 32 bits |
E = Exp - Bias
- Exp: unsigned value of exp field.
- Bias = , k is number of exp’s bits
- Single precision:
- Double precision:=1023
M=,there first 1 is not stored.
Example
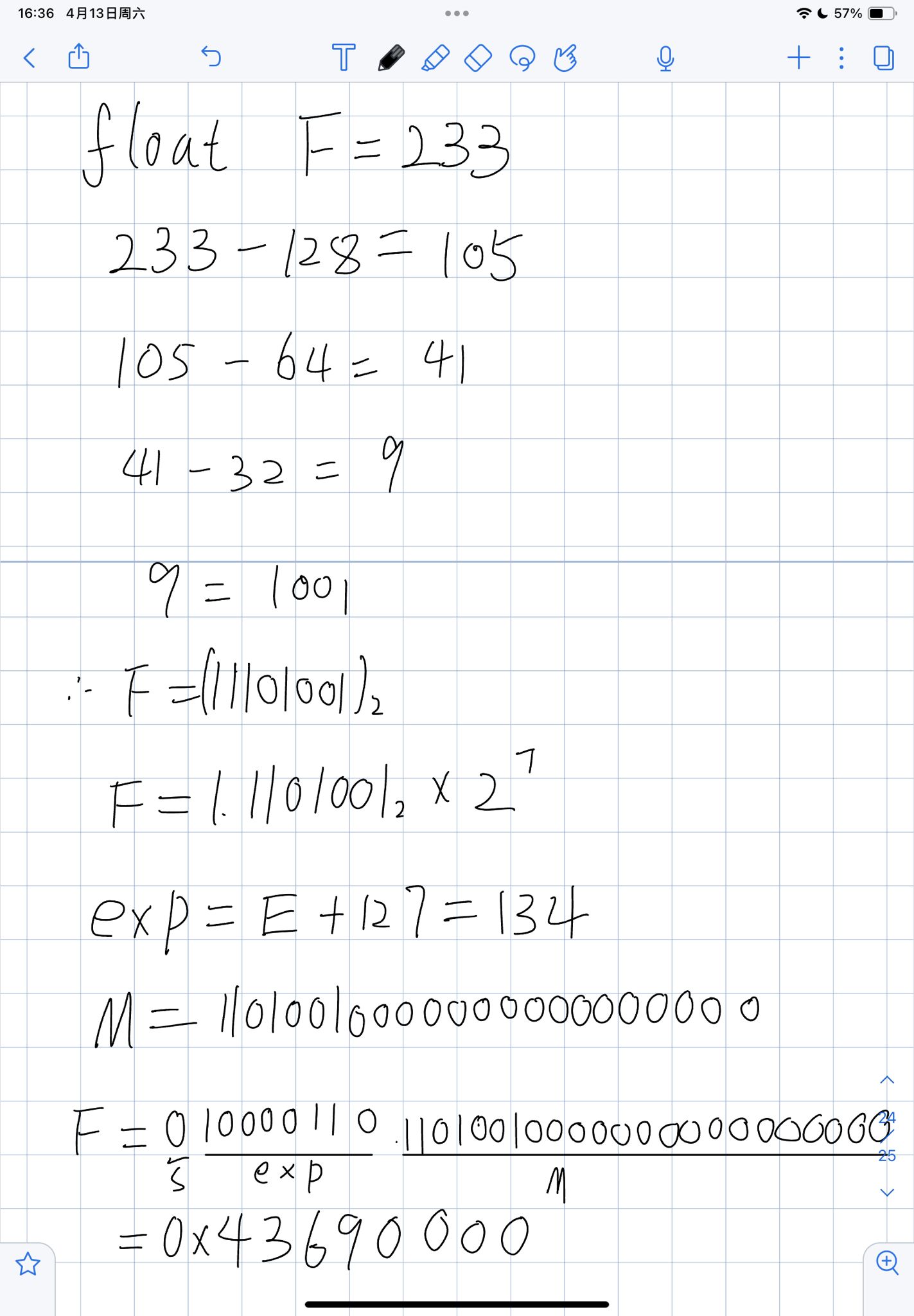
Denormalized Values
Condition:exp=000…0
E=1-Bias(instead of E = 0-Bias)
Significand coded with implied leading 0: M =
it’s usually represents closest to 0.0
exp = 0, frac = 0, Represents zero value.
Special Values
Condition:exp=111…1
Case:exp=111…1, frac = 0, infinity,E.g.1.0/0.0=-1.0/-0.0=positive infinity,1.0/-0.0=negative infinity
Case:exp=111…1,frac!=0, NaN
Round-To-Even
When exactly halfway between two possible values,Round so that least significant digit is even
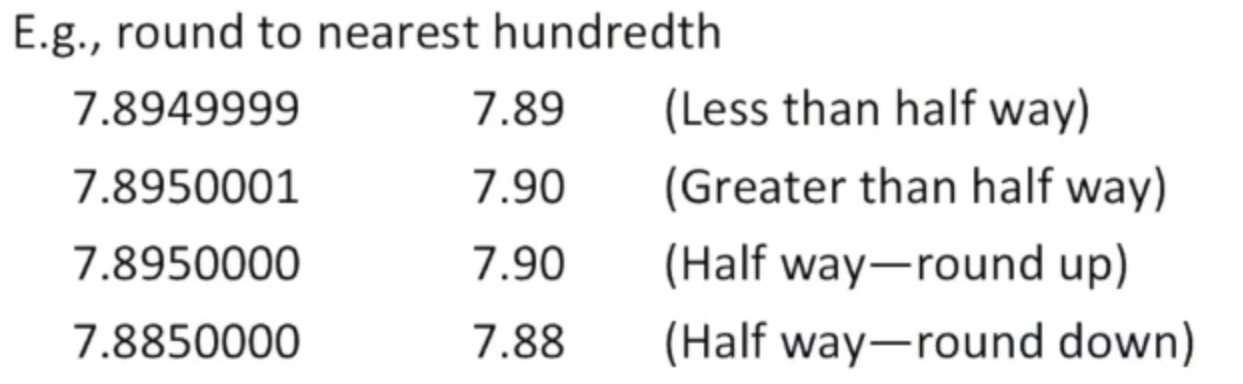
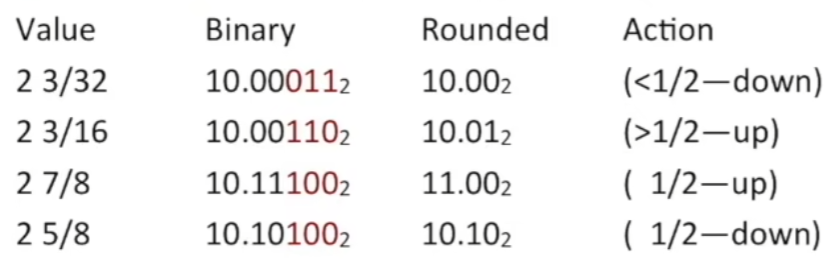
Multiplication
Exact Result:
- Sign s : ^
- Significand M :
- Exponent E :
if M>=2,shift M right, increment E
if E out of range, oveflow
Round M to fit frac precision
Addition
Exact Result:
- E :
- M : line up the binary points and add
float point addition is not commutative compliance law
1 | (3.14+1e10)-1e10=0, 3.14+(1e10-1e10)=3.14 |
(update date:2024/4/13)
Machine-Level Programming I: Basics
Assembly/Machine Code View
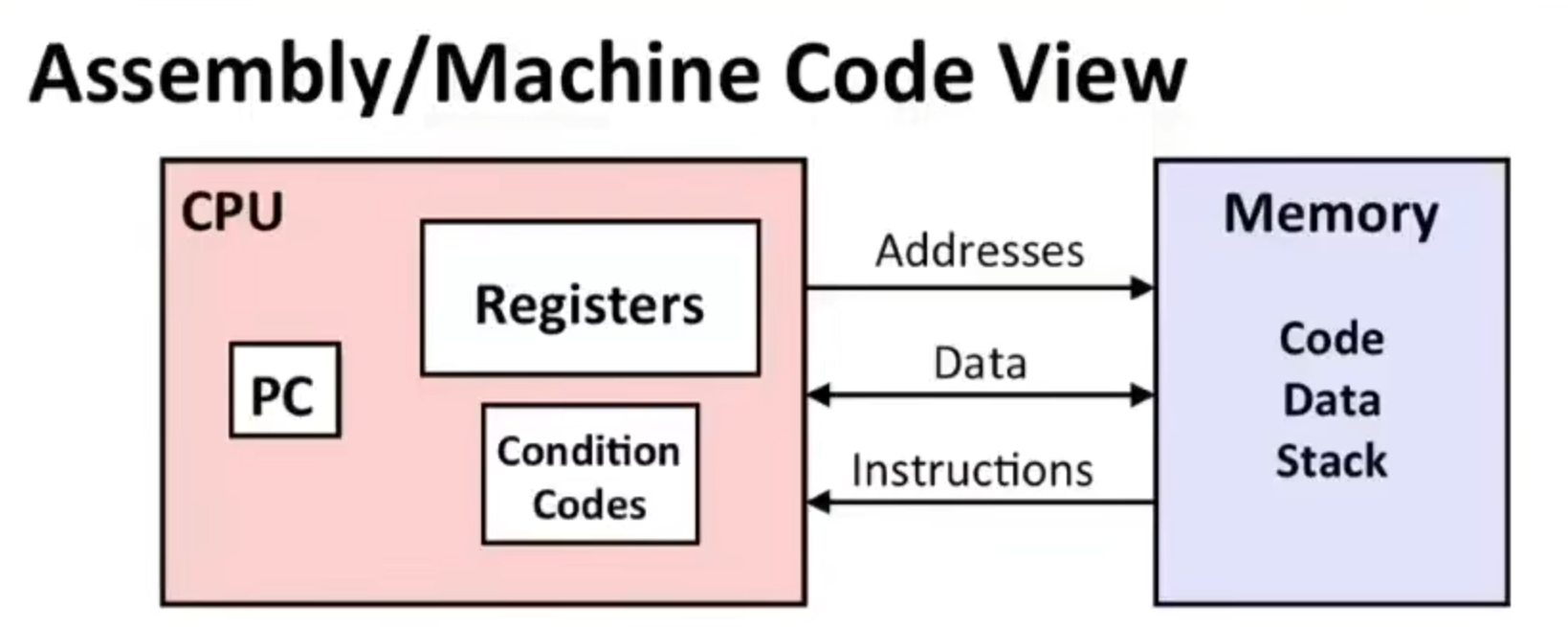
PC:Program counter
- Address of next instruction
- Called “RIP”(x86-64)
Register file
- Heavily used program data
Condition codes
- Store status information about most recent arithmetic or logical operation
- used for conditional branching
Memory
- Byte addressable array
- Code and user data
- Stack to support procedures
Assembly Characteristics
DataType
integer data of 1, 2, 4, 8bytes
- Data values
- Addresses(untyped pointers)
Floating point data of 4, 8, or 10 bytes
Code:Byte sequences encoding series of instructions
Arrays or structures: just contiguously allocated bytes in memory
Operations
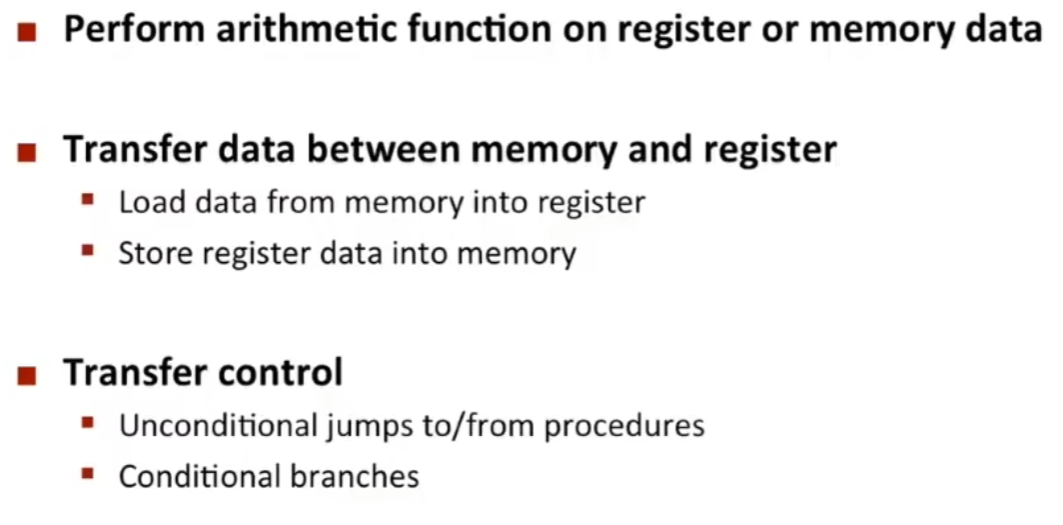
Example
C : store value t where designated by dest
1 | *dest = t; |
Assembly : Move 8 byte to memory.
1 | moveq %rax, (%rbx) |
Object Code: 3-byte instruction stored at address 0x40059e
1 | 0x40059e: 48 89 03 |
Disassembly
We can use gdb to get assembly.
1 | gdb ./bomb |
also, We can get same bytes on a address.like this, I can get string by address.(/s is ask to gdb use which way to get bytes)
X86-64 Interger Registers
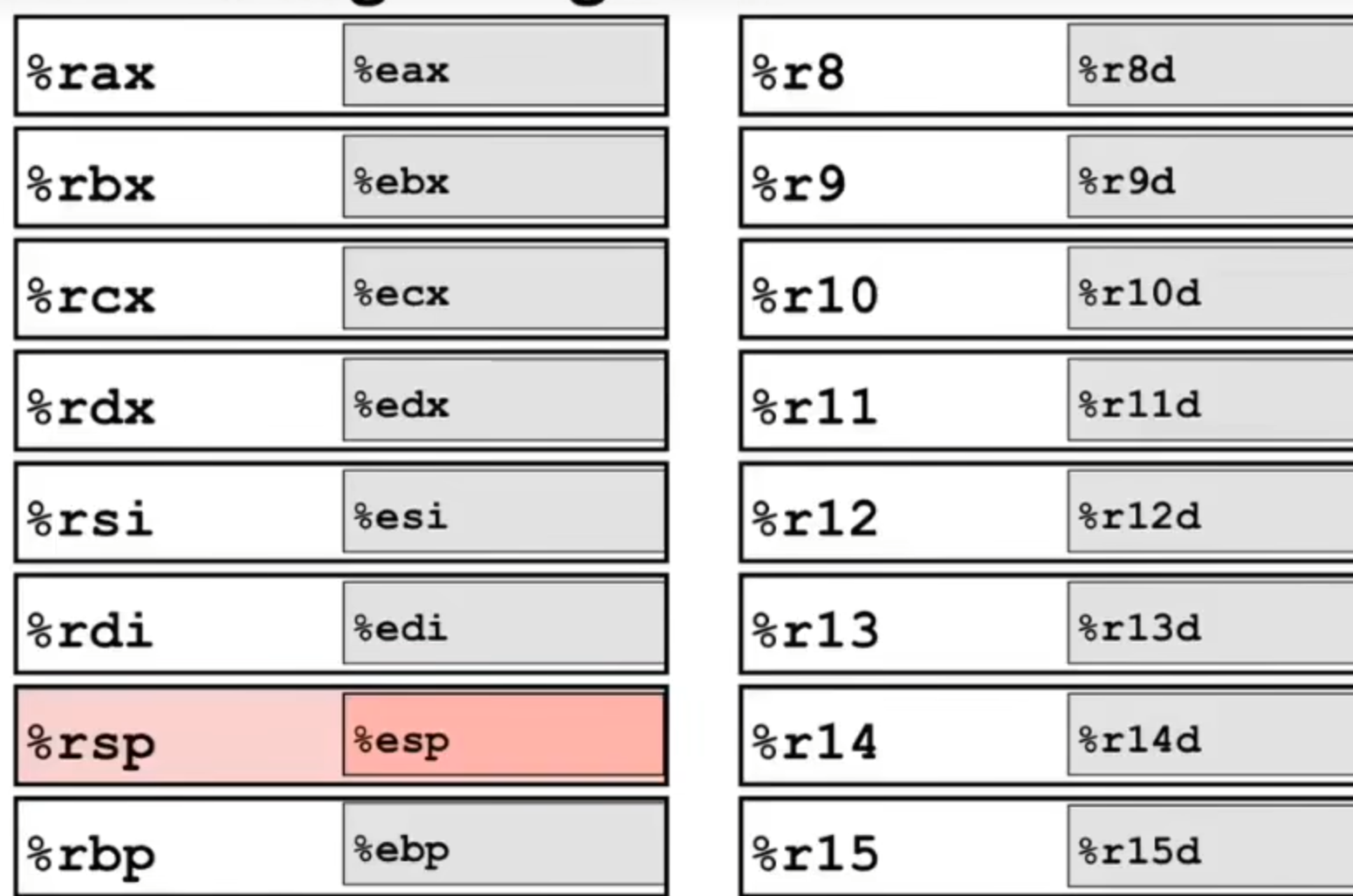
Can reference low-order 4 bytes (also low-order 1 & 2 bytes)
Moving Data
movq Source, Dest;
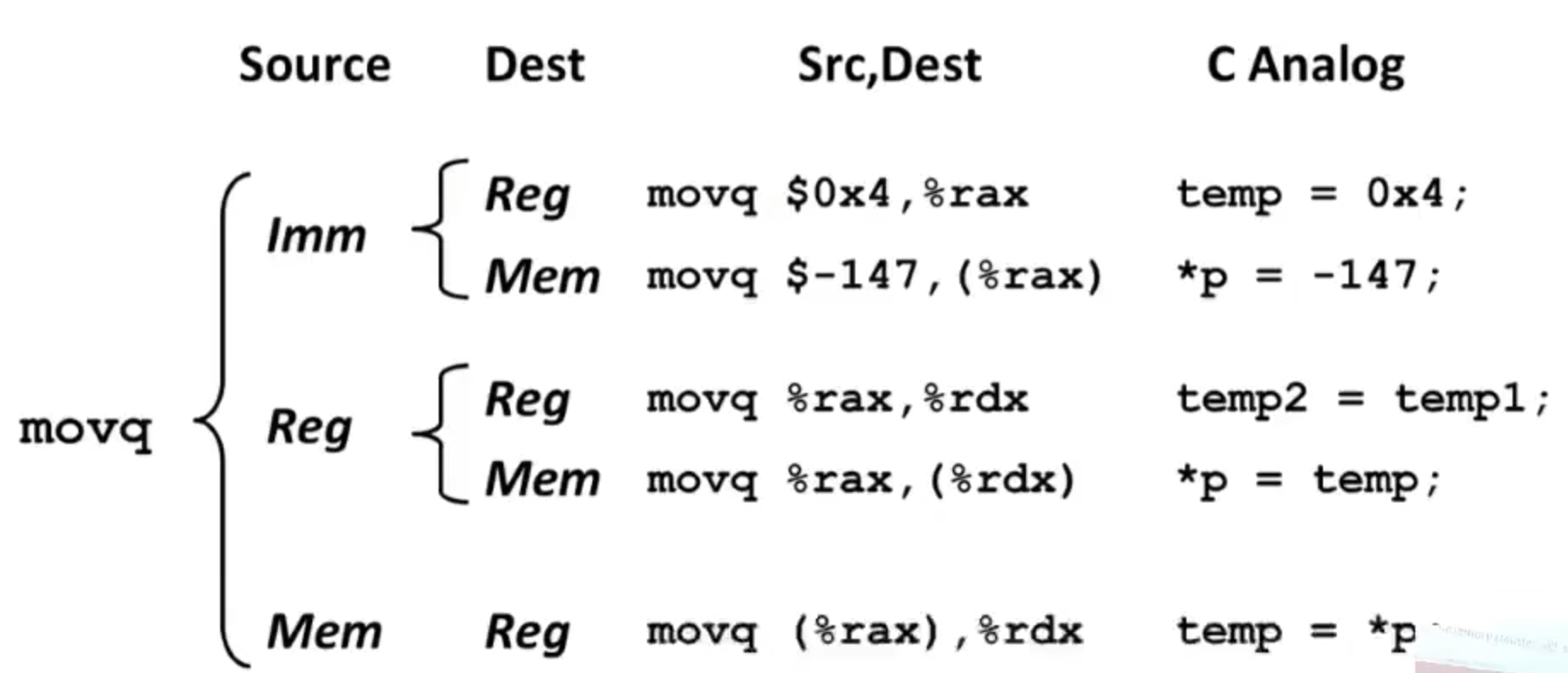
Cannot do memory-memory transfer with a single instruction.
example
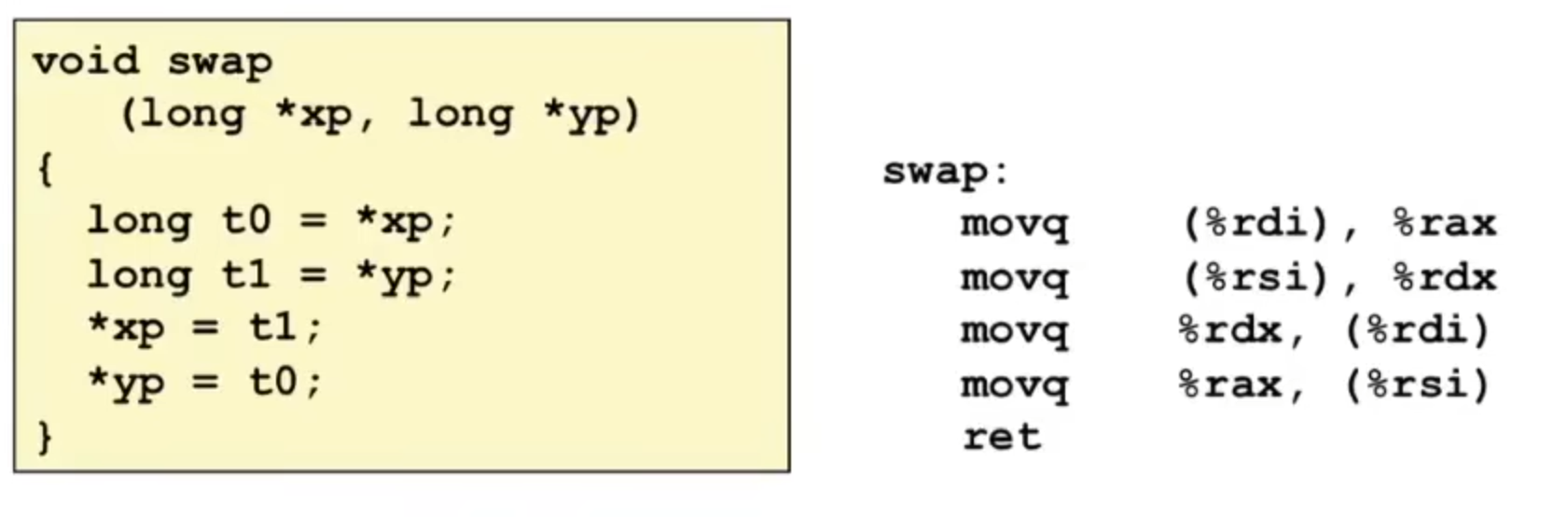
Most General Form
D(Rb,Ri,S) Mem[Reg[Rb]+S*Reg[Ri]+D]
D: Constant “displacement” 1, 2 or 4 bytes
Rb: Base register: Any of 16 integer registers
Ri: index register: Any, except for %rsp
S: Scale: 1,2,4, or 8
Address Computation Instruction
leaq Src, Dst
- Src is address mode expression
- Set Dst to address denoted by expression
1 | long m12(long x) { |
Some Arithmetic Operations
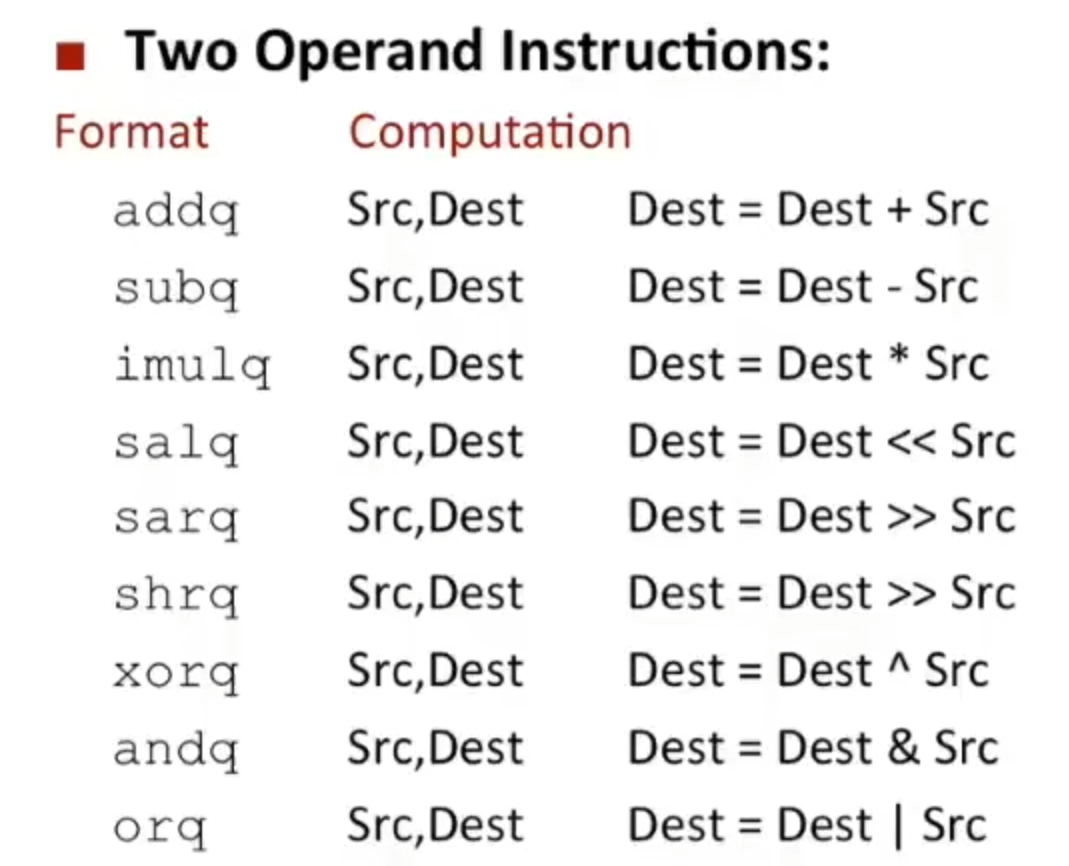
(update date: 2024/4/14)